Fortunately, there are many Python utilities that help convert files from .py to .exe. They will “freeze” your code and build an executable file for you. Here we’ll consider two of the most popular options: cx_freeze and PyInstaller. Both of these tools are versatile and easy to use, but depending on your use case, you may prefer one over the other.
Installing Python
If you already have Python installed, you can skip this step. However, for those who haven’t, read on.
For this tutorial you can use ActiveState’s Python, which is built from vetted source code and regularly maintained for security clearance. You have two choices:
- Download and install the pre-built “Python Executable” runtime environment for Windows 10 or CentOS 7, or…
- Build your own custom Python runtime with just the packages you’ll need for this project, by creating a free ActiveState Platform account, after which you will see the following image:
How to Install Python Packages with the ActiveState Platform
- Click the Get Started button and choose Python and the OS you’re working in. Choose the packages you’ll need for this tutorial, including cx_freeze and PyInstaller.
- Once the runtime builds, you can download the State Tool and use it to install your runtime.
And that’s it! You now have installed Python in a virtual environment.
.py to .exe: How to Create an .exe file From a .py File
We’ll need a Python program from which to build our executable. For this example, I’m using a simple script that prints out Pascal’s Triangle, named pascals_triangle.py
pascals_triangle.py --------------------------------- import time n=int(input("Enter number of rows: ")) a=[] for i in range(n): a.append([]) a[i].append(1) for j in range(1,i): a[i].append(a[i-1][j-1]+a[i-1][j]) if(n!=0): a[i].append(1) for i in range(n): print(" "*(n-i),end=" ",sep=" ") for j in range(0,i+1): print('{0:6}'.format(a[i][j]),end=" ",sep=" ") print() time.sleep(500) --------------------------------------------------
Assuming you’re using a Windows machine, all you need to do to build an .exe file from pascals_triangle.py is:
- Open up the command line
- Navigate to the folder that contains the pascals_triangle.py program
- Run
pyinstaller pascals_triangle.py
This creates a folder called “dist” in the folder that contains your Python code, and within that folder you’ll find your .exe file.
This process is almost as simple with cx_freeze, but requires an extra configuration step. Before running cx_freeze, you’ll need to create a file called “setup.py” that is stored in the same folder as the pascals_triangle.py program. The setup.py file configures the options for cx_freeze, and can get complicated if you’re trying to do something very particular. For this example, however, it’s fairly simple:
setup.py ---------------------------------------------------------- from cx_Freeze import setup, Executable setup(name = "pascals_triangle" , version = "0.1" , description = "" , executables = [Executable("pascals_triangle.py")]) ----------------------------------------------------------
To run cx_freeze, you can now:
- Navigate to the folder containing pascals_triangle.py and setup.py
- Run the command
python setup.py build
When cx_freeze is done running the .exe file will be available in a directory within the folder that contains setup.py.
Simplicity vs. Configurability
While the setup file required by cx_freeze presents an additional step in the process, it also enables great flexibility. In the setup file you can specify which modules, files, and packages to include (or exclude) from the build. You can set a target name for your executable, as well as a path to the icon that should be used to represent it. There’s also an option to set chosen environment variables upon installation. All this helps you to deliver an executable that matches your needs precisely.
Conversely, PyInstaller’s approach is to let you just freeze your code without worrying about all the options. It supports most libraries out-of-the-box, so the hassle of including dependent libraries is already taken care of. For the most common use cases, PyInstaller is all you need. However, if you find that you have something like hidden imports to deal with, it’s much trickier to specify these requirements in PyInstaller.
Cross-Platform Compatibility
Both cx_freeze and PyInstaller are cross-platform in the sense that they can be used on any OS. However, neither of them have the ability to cross-compile. So if you’re using either tool on a Mac, you’ll be able to generate .app files but not .exe. In other words, if you need to distribute your program to users on a variety of platforms, you’ll need to use a variety of platforms to build them.
Code Obfuscation
Both cx_freeze and PyInstaller build a distribution that is free from your original source code. However, both include compiled Python scripts (.pyc files). These could, in principle, be decompiled to reveal the logic of your code. If you want to protect your app against being reverse-engineered, you can encrypt the bytecode. PyInstaller provides a command line argument to obfuscate the bytecode with the Advanced Encryption Standard, AES256. In order to accomplish the same thing with cx_freeze, you’ll need to install an additional tool such as pyarmor or pyobfuscate.
One-file Mode
The motivation for this process is to make things easy for the users of your application. So what could be easier than giving them a single executable file to click on? Collapsing the entire directory into a single file is an option in PyInstaller, though not in cx_freeze. If your end-users won’t be comfortable without the push-button simplicity of a single .exe file, then this feature alone makes PyInstaller the clear choice.
Converting .py to .exe: Conclusions
Pros | Cons | |
PyInstaller | – Simple to use; no configuration required – Built-in code obfuscation – Can reduce directory to single executable |
– More difficult to customize – Cannot cross-compile |
cx_freeze | – Highly configurable – Easy to explicitly include or exclude libraries – Can set environment variables on installation |
– More complicated to use – Cannot cross-compile – Executable must be distributed as part of a larger directory |
For applications that rely on the most common libraries, PyInstaller will work right out-of-the-box and deliver the executable you need. Its simplicity, combined with some very useful features, make it a good first choice in most cases. However, if you find that your source code requires some more careful configuration, cx_freeze provides more options and explicit control when trying to convert .py to .exe.
Ultimately, both tools are easy to use and will convert your python code to an executable file that you can share. Whether or not your application is truly ready for distribution is up to you!
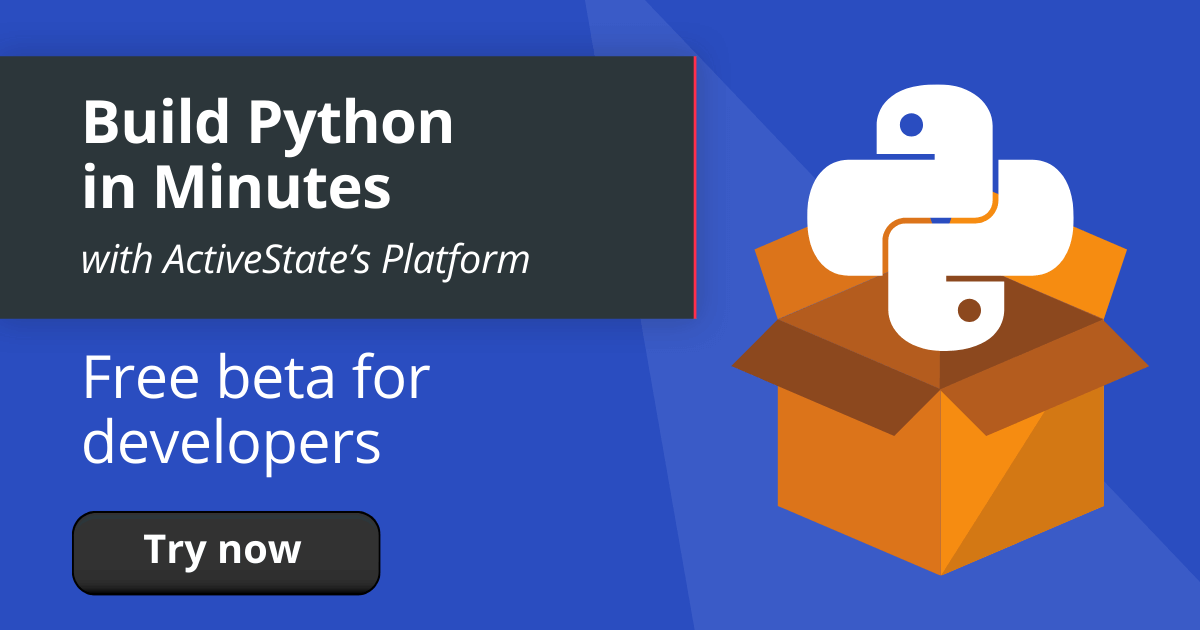
Download and install the pre-built “Python Executable” runtime runtime environment for Windows 10, macOS, CentOS 7, or automatically build your own custom Python runtime on the ActiveState Platform
Frequently Asked Questions
How do I convert Python to .exe?
Get both cx_freeze and PyInstaller in our pre-built Python Executable environment.
Does Python convert files to .exe on its own?
You can customize a Python runtime environment with both standard and third-party libraries/packages with a free account on the ActiveState Platform.
How do I create a Python .exe file for Windows?
Can I double click a .py file to run it without converting to .exe?
Download our pre-built Python Executable environment, which includes both cx_freeze and PyInstaller.