Imagine living in a world where you could easily access anything sensitive with just a glance – a world where the use of facial verification and recognition technology was integrated into our daily lives. All of this is possible using Python, as long as it’s done correctly and with the right security measures in place.
What Is Facial Recognition?
Although the terms might seem similar, facial recognition and face verification are not the same:
- Facial Recognitionis the process of identifying an individual by comparing an image of his or her face to multiple images of faces in a database.
- It’s like a one-to-many comparison, where the image is compared to several possible candidates. It answers the question, “who is this person?”
- Face Verificationis the process of determining whether a set of faces match each other. It’s more like comparing a given face to a known reference (in many cases, this reference is a database of stirred images). It’s used to determine if the face in the images belongs to the same individual.
- It’s more like a one-to-one comparison, and it answers the question, “is this person who he or she claims to be?”
Simply put, face verification confirms an individual’s identity, while facial recognition identifiesa person from a database of known people. These two methods can be used hand-in-hand to develop a complete, customizable system.
In this tutorial, we will walk you through a step-by-step guide to building a face verification system using Python. We’ll also explain how to gather data for training purposes, and show you how to test it. The steps we’ll follow include:
- Installing a Python virtual environment with all the packages we’ll need
- Writing a Face Verification program and testing it out
- Dealing with limitations
Finally we’ll consider how our solution compares to some other biometric security measures. Put on your tech hat and let’s start writing some code!
Step 1 — Install Face Verification Python Environment
To follow along with the code in this article, you can download and install our pre-built Face Verification Python environment, which contains:
- A version of Python 3.10.
- All the dependencies used in this post in a pre-built environment for Windows and Linux.
In order to download this ready-to-use Python project, you will need to create a free ActiveState Platform account. Just use your GitHub credentials or your email address to register. Signing up is easy and it unlocks the ActiveState Platform’s many other dependency management benefits.
Or you can also use our State tool CLI to install the Face Recognition runtime environment:
Windows users can install the Face Verification runtime into a virtual environment by downloading and double-clicking on the installer. You can then activate the project by running the following command at a Command Prompt:
state activate --default Pizza-Team/Face-Verification
For Linux users, run the following to automatically download, install and activate the Face Verification runtime in a virtual environment:
sh <(curl -q https://platform.activestate.com/dl/cli/_pdli01/install.sh) -c'state activate --default Pizza-Team/Face-Verification
Step 2 — Building a Face Verification Program
Once the runtime environment is installed, you can set up your IDE. If you use Visual Studio Code (VS Code) you can set it up to work with the ActiveState interpreter by simply selecting it from the interpreters dropdown.
We can test to make sure that our libraries were correctly installed into our development environment by importing them into our Python script (the file where we are going to write our code):
import cv2 import face_recognition import numpy as np
If no errors are encountered, that means our development environment is ready for use. If there are errors, it will usually be because you are in the wrong development environment or because the imported libraries were not installed properly (in which case they will be underlined in red).
Loading an Image in Python
We begin by loading a known face image (such as one of your own face) using the face_recognition.load_image_file() and encoding it. The encoding is saved in the first available slot (which is indicated by [0]):
# Load a known face image known_image = face_recognition.load_image_file("known_face.jpg") known_face_encoding = face_recognition.face_encodings(known_image)[0]
Initialize Camera Capture in Python
Initialize the webcam using cv2.VideoCapture.
# Initialize the webcam video_capture = cv2.VideoCapture(0)
Looping in Python
We then begin a “while” loop that captures frames from the webcam using the video_capture.read() function. For all frames that are captured, it searches for the location of the face and encodes it correctly.
Another round of looping is performed on each face it finds. The encoding is compared to that of the known face loaded earlier. If there is a match, it sets the label to “Pass,” but if the two faces don’t match, then it displays the “Fail” label:
while True: # Capture a frame from the webcam ret, frame = video_capture.read() # Find all face encodings in the frame face_locations = face_recognition.face_locations(frame) face_encodings = face_recognition.face_encodings(frame, face_locations) # Loop through each face found in the frame for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings): # Compare the face encoding with the known face encoding matches = face_recognition.compare_faces([known_face_encoding], face_encoding) # Set the label to "Fail" by default label = "Fail" # If a match is found, set the label to "Pass" if True in matches: label = "Pass" # Draw a rectangle around the face and display the label cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2) cv2.putText(frame, label, (left + 6, bottom - 6), cv2.FONT_HERSHEY_DUPLEX, 0.5, (255, 255, 255), 1) # Display the resulting frame cv2.imshow("Face Recognition", frame)
Exiting a Python Loop
To exit the loop, we can have the user press the letter q. At this point, the webcam is released and all windows are closed.
# Exit the loop if the 'q' key is pressed if cv2.waitKey(1) & 0xFF == ord("q"): break # Release the webcam and close all windows video_capture.release() cv2.destroyAllWindows()
Testing Facial Verification in Python
A successful test of the program is composed of:
- Opening a window that displays your webcam feed. Faces will be detected in real time and compared to the known faces that you have in your database.
- If a face is recognized, the “Pass” label will be shown; otherwise, the “Fail” label will be displayed.
- In order to exit the program, press q and the program will stop.
Step 3 — Challenges and Limitations of Facial Recognition
Although the use of face verification as a method of authentication is increasing, there are still a number of challenges and limitations associated with this method. Some of the most common ones include:
- Lighting conditions:Light is one of the major issues that might affect the functioning of such a program. Situations in which there are shadows, overexposures, or insufficient lighting can negatively affect performance. To help correct such issues, try working with a training dataset that includes diverse lighting conditions.
- Pose variations: Different head poses might also affect the system’s ability to verify someone’s identity. To be sure that you counter this problem at an early stage, experiment with different poses, or use trained models that are able to capture faces at all angles.
- Aging: Facial features change as people age, which can affect the accuracy of face verification systems. To address this issue, consider using a more dynamic dataset, or seasonally updating the reference images to ensure the system adapts to the user’s changing appearance.
Step 4 — Comparison of Biometric Authentication Methods
Each biometric method has its own strengths and weaknesses, and choosing the right one for your needs is very important. We have discussed some of the uses of facial technology and why its adoption is on the rise, but how does it compare with other biometric methods?
Fingerprinting— one of the oldest and most widely used methods of authentication since its pros typically outweigh its cons for most use cases:
- Pro – high accuracy, because each individual has unique fingerprint patterns that remain unchanged throughout their lifetime.
- Pro – low cost, since fingerprint scanners are relatively cheap and easy to integrate.
- Pro – fast, making it suitable for real-time applications.
- Con – vulnerable to spoofing, as the sensors can be easily tricked using fake prints made from material like silicone.
- Con– need for physical contact with the scanner raises hygiene issues, especially in public places or on shared devices.
Iris Scanners – examine unique patterns found in the colored part of the eye to verify an individual’s identity.
- Pro – extremely accurate.
- Pro – eliminates hygiene concerns since it’s contactless.
- Pro – performance is consistent since the iris remains unchanged throughout a person’s lifetime.
- Con – more expensive to implement compared to facial verification or fingerprint systems.
- Con– users may find it uncomfortable to align their eyes with the scanners, especially those with vision impairments.
Voice Recognition – uses the unique characteristics of a person’s voice to identify them or verify their identity.
- Pro – convenience, since it can be performed remotely and does not require any specialized hardware.
- Pro– highly flexible, and can be integrated into various applications (such as virtual assistants, smartphone devices, and telephone banking).
- Con – a person’s voice can change due to different factors, which will impact accuracy rates.
- Con – far too easy to spoof with high-quality voice recordings or synthesized speech.
Facial verification is a convenient, contactless solution for identity verification , but depending on your use case, one of the other methods listed above may be more suitable.
Conclusions – Python for Face Verification Use Cases
With the wide usage of smart devices with integrated cameras, face verification has become extremely popular, and is now the go-to method for securing data and devices. As a result, the simple program we’ve written in this tutorial can be applied to a wide range of use cases, including:
- Smartphone authentication– Although other methods are still in use (like patterns and fingerprints), many modern devices have adopted facial recognition as an authentication method.
- Access control– Many corporate buildings, educational institutions, and other facilities have adopted face verification to grant access based on an individual’s identity.
- Law enforcement and border control– With the increase in crime and movement across borders, government authorities have adopted facial verification to confirm the identity of travelers or suspects using a database of known individuals. This helps them ensure and maintain a higher level of security.
- Financial services– As an extra security measure, banks and financial institutions have started to integrate face verification into their mobile applications and ATM machines, providing a more secure way to perform transactions.
In conclusion, facial recognition is a useful and valuable method of verification. Although it has some limitations in terms of security, these concerns can be addressed by adopting:
- Enhanced algorithms– Using enhanced techniques from machine learning and deep learning, we can get better results from facial feature extraction and comparison algorithms. This will increase accuracy and reduce false verification.
- Liveness detection– The integration of liveness detection can help prevent spoofing attacks by ensuring only live faces are authenticated (rather than images).
- Integration with other biometric techniques – Combining facial verification with other biometric methods can help enhance security and accuracy.
By staying up to date with the latest developments, it is possible to harness the potential of facial verification for numerous applications.
Next steps:
Sign up for a free ActiveState Platform account and download the Face Verification Python environment so you can create your own facial recognition program.
.
Read Similar Stories
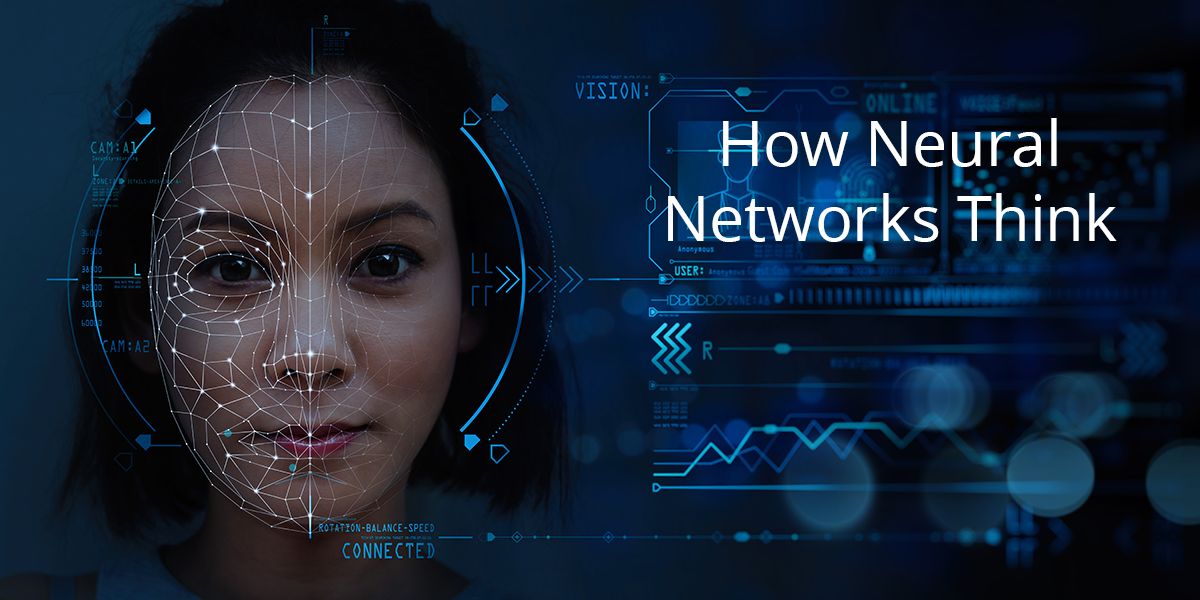
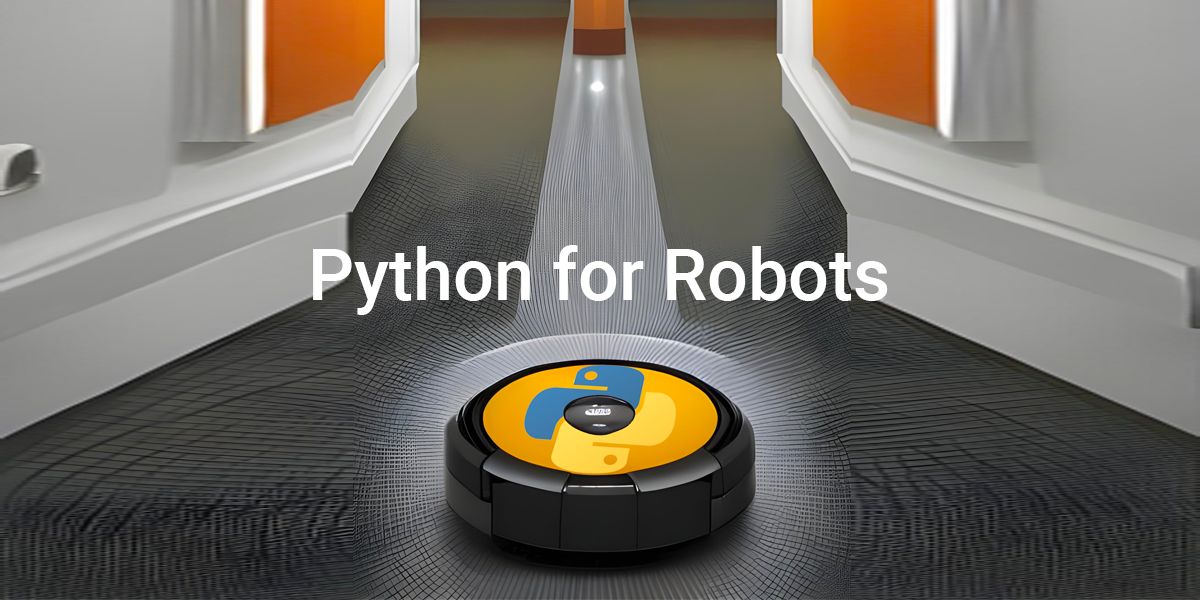